Hi Techies!
In a nutshell, the execution context represents the time between when the code is executed and when it ends. The important thing to understand is that the Apex code is not always the only code that is executing. Confusing right? Don’t worry let’s see all the ways Apex code can be executed(or invoked) on the Salesforce platform.
Method | Description |
---|---|
Database Trigger | Invoked for a specific event on a custom or standard object. |
Anonymous Apex | Code snippets executed on the fly in Dev Console & other tools. |
Asynchronous Apex | Occurs when executing a future or queueable Apex, running a batch job, or scheduling Apex to run at a specified interval. |
Web Services | Code that is exposed via SOAP or REST web services. |
Email Services | Code that is set up to process inbound email. |
Visualforce or Lightning Pages | Visualforce controllers and Lightning components can execute Apex code automatically or when a user initiates an action, such as clicking a button. Lightning components can also be executed by Lightning processes and flows. |
Apart from this, some actions as creating a new task, sending an email, performing a field update, etc. can be triggered by one of the declarative platform features. These actions also run within an execution context.
For example, if you run an apex code like a trigger in the Anonymous window and open the execution log, it looks something like this:
Notice that the first line in the execution log marks the EXECUTION_STARTED event and that the last line is the EXECUTION_FINISHED event. Everything in between is the execution context.
A CODE_UNIT_STARTED event marks when the code from the Execute Anonymous window is kicked off. This line is highlighted in red in the image below.
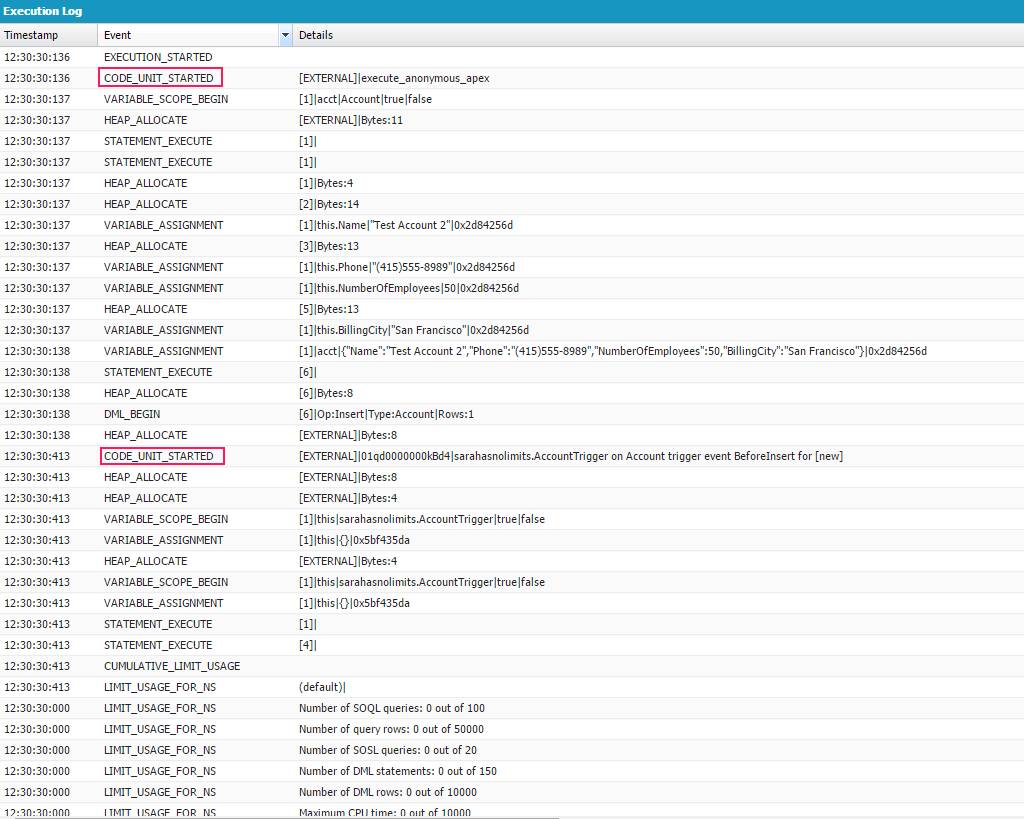
The second CODE_UNIT_STARTED line that is highlighted represents when code for something like a BeforeInsert event was executed.
How to get the current Execution Context using Apex:
We can get the current execution context at runtime using the Request class which contains methods to obtain the request ID and Quiddity value of the current Salesforce request.
The methods in the Request class obtain a unique request ID and the Quiddity value that represents the current Apex execution type.
Example:
//Get info about the current request
Request reqInfo = Request.getCurrent();
//Get the identifier for this request, which is universally unique
String currentRequestId = reqInfo.getRequestId();
//Enum representing how Apex is running. e.g. BULK_API vs LIGHTNING
Quiddity currentType = reqInfo.getQuiddity();
Quiddity is an ENUM with values like ANONYMOUS,BATCH_APEX etc.
Complete values of Quiddity here: https://developer.salesforce.com/docs/atlas.en-us.apexref.meta/apexref/apex_enum_System_Quiddity.htm?q=Quiddity
Why do we need to care about Execution context?
It is important to understand the concept of an execution context because salesforce limits are tied to each of these contexts.